[A-00093]pythonでNatural Language API(GCP)を使用する
Google Cloudが提供するNatural Language APIをpythonで使用する方法を記載する。
公式ドキュメントは下記
https://cloud.google.com/natural-language?hl=ja
・セットアップ
pythonライブラリをインストールします。
pip install --upgrade google-cloud-language
・簡単な感情分析を行う
お決まりの[Hello,world!]をAPIに読み込ませてみます。
# Imports the Google Cloud client library
from google.cloud import language_v1
# Instantiates a client
client = language_v1.LanguageServiceClient()
# The text to analyze
text = "Hello, world!"
document = language_v1.types.Document(
content=text, type_=language_v1.types.Document.Type.PLAIN_TEXT
)
# Detects the sentiment of the text
sentiment = client.analyze_sentiment(
request={"document": document}
).document_sentiment
print(f"Text: {text}")
print(f"Sentiment: {sentiment.score}, {sentiment.magnitude}")
(.venv)MacBook-Pro:test2_project$ python3 languagetest.py
Text: Hello, world!
Sentiment: 0.6000000238418579, 0.6000000238418579
・文章の分析を行う
次に文章の分析を行います。
サンプルテキストは下記のとおりです。
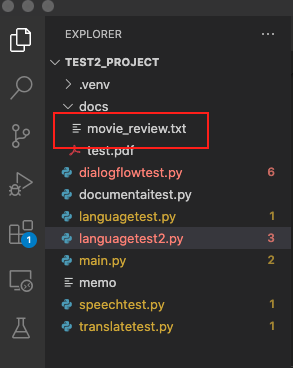
中身は英語ですが、「退屈」「感傷的」「とても悪い」などのネガティブワードを入れています。
import argparse
from google.cloud import language_v1
def print_result(annotations):
score = annotations.document_sentiment.score
magnitude = annotations.document_sentiment.magnitude
for index, sentence in enumerate(annotations.sentences):
sentence_sentiment = sentence.sentiment.score
print(f"Sentence {index} has a sentiment score of {sentence_sentiment}")
print(f"Overall Sentiment: score of {score} with magnitude of {magnitude}")
return 0
def analyze(movie_review_filename):
"""Run a sentiment analysis request on text within a passed filename."""
client = language_v1.LanguageServiceClient()
with open(movie_review_filename) as review_file:
# Instantiates a plain text document.
content = review_file.read()
document = language_v1.Document(
content=content, type_=language_v1.Document.Type.PLAIN_TEXT
)
annotations = client.analyze_sentiment(request={"document": document})
# Print the results
print_result(annotations)
if __name__ == "__main__":
parser = argparse.ArgumentParser(
description=__doc__,
formatter_class=argparse.RawDescriptionHelpFormatter
)
parser.add_argument(
"movie_review_filename",
help="The filename of the movie review you'd like to analyze.",
)
args = parser.parse_args()
analyze(args.movie_review_filename)
python3 languagetest2.py ./docs/movie_review.txt
(.venv)MacBook-Pro:test2_project$ python3 languagetest2.py ./docs/movie_review.txt
Sentence 0 has a sentiment score of -0.800000011920929
Sentence 1 has a sentiment score of -0.6000000238418579
Sentence 2 has a sentiment score of -0.800000011920929
Overall Sentiment: score of -0.800000011920929 with magnitude of 2.4000000953674316
上記の通り、感情分析を数値で表示してくれました。ちなみに表示は行ごとに行われるようです。
コメントを残す