[A-00092]pythonでDocument AI API(GCP)を使用する
Google Cloudが提供するDocument AI APIをpythonから使用する方法を記載する。
公式ドキュメントはこちら
https://cloud.google.com/document-ai/docs/libraries
・セットアップ
pythonのライブラリをインストールします。
pip install --upgrade google-cloud-documentai
・Document AIを使用する前にProcessor-Typeを調べる
Document AIを使用する前に自分の使用するProcessor Typeを決める必要があります。
Processor-Typeを調べる方法は下記のとおりです。
from google.api_core.client_options import ClientOptions
from google.cloud import documentai # type: ignore
if __name__ == "__main__":
client = documentai.DocumentProcessorServiceClient()
# Initialize request argument(s)
request = documentai.FetchProcessorTypesRequest(
parent="projects/<project_id>/locations/us",
)
# Make the request
response = client.fetch_processor_types(request=request)
print(response)
上記で取得したProcessor-Typeから使用するドキュメントに合わせて選択して下さい。
今回の場合はPDFファイルなので[OCR_PROCESSOR]で読み取りします。
・ドキュメントを読み込む(新規Processorを作成する)
使用するドキュメントを下記のように適当なローカルフォルダに格納してください。
今回テスト用で使用するファイルは下記です。
下記のようにローカルの適当なディレクトリに格納してください。
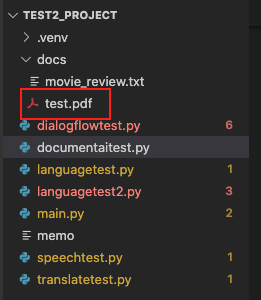
from google.api_core.client_options import ClientOptions
from google.cloud import documentai # type: ignore
def quickstart(
project_id: str,
location: str,
processor_display_name: str,
processor_type: str,
file_path: str,
mime_type: str,
):
# You must set the `api_endpoint`if you use a location other than "us".
opts = ClientOptions(api_endpoint=f"{location}-documentai.googleapis.com")
client = documentai.DocumentProcessorServiceClient(client_options=opts)
# The full resource name of the location, e.g.:
parent = client.common_location_path(project_id, location)
# Create a Processor
processor = client.create_processor(
parent=parent,
processor=documentai.Processor(
display_name=processor_display_name, type_=processor_type
),
)
# Print the processor information
print(f"Processor Name: {processor.name}")
# Read the file into memory
with open(file_path, "rb") as image:
image_content = image.read()
# Load binary data
raw_document = documentai.RawDocument(content=image_content, mime_type=mime_type)
# Configure the process request
request = documentai.ProcessRequest(name=processor.name, raw_document=raw_document)
result = client.process_document(request=request)
document = result.document
# Read the text recognition output from the processor
print("The document contains the following text:")
print(document.text)
# [END documentai_quickstart]
return processor
def main():
quickstart("<project_id>"
, "us"
, "MyProcessorsOCR"
, "OCR_PROCESSOR"
, "<path_to_pdf>/test.pdf"
, "application/pdf")
if __name__ == "__main__":
main()
# end main
(.venv) MacBook-Pro:test2_project$ python3 documentaitest.py
Processor Name: projects/xxxxxxx/locations/us/processors/xxxxxxx
The document contains the following text:
備考欄
下記のとおりご請求申し上げます。
お振込先
お支払い期限
御中
品名
請求書
0
数量 単位
所在地:
TEL:
担当:
請求書発行日:
請求番号:
円 (消費税込)
単価
小 計
税率
消費税
合計
金額
10%
0
0
0
0
0
0
0
0
確定
上記の通り、Document AIでpdfファイルがうまく読み込めました。
・カスタムプロセッサのAPIでドキュメントを分析する。
詳しくは下記の記事に書いてます。
ソースコードは下記です。
from typing import Optional
from google.api_core.client_options import ClientOptions
from google.cloud import documentai # type: ignore
# TODO(developer): Uncomment these variables before running the sample.
# project_id = "YOUR_PROJECT_ID"
# location = "YOUR_PROCESSOR_LOCATION" # Format is "us" or "eu"
# processor_id = "YOUR_PROCESSOR_ID" # Create processor before running sample
# file_path = "/path/to/local/pdf"
# mime_type = "application/pdf" # Refer to https://cloud.google.com/document-ai/docs/file-types for supported file types
# field_mask = "text,entities,pages.pageNumber" # Optional. The fields to return in the Document object.
# processor_version_id = "YOUR_PROCESSOR_VERSION_ID" # Optional. Processor version to use
def process_document_sample(
project_id: str,
location: str,
processor_id: str,
file_path: str,
mime_type: str,
field_mask: Optional[str] = None,
processor_version_id: Optional[str] = None,
) -> None:
# You must set the `api_endpoint` if you use a location other than "us".
opts = ClientOptions(api_endpoint=f"{location}-documentai.googleapis.com")
client = documentai.DocumentProcessorServiceClient(client_options=opts)
if processor_version_id:
# The full resource name of the processor version, e.g.:
# `projects/{project_id}/locations/{location}/processors/{processor_id}/processorVersions/{processor_version_id}`
name = client.processor_version_path(
project_id, location, processor_id, processor_version_id
)
else:
# The full resource name of the processor, e.g.:
# `projects/{project_id}/locations/{location}/processors/{processor_id}`
name = client.processor_path(project_id, location, processor_id)
# Read the file into memory
with open(file_path, "rb") as image:
image_content = image.read()
# Load binary data
raw_document = documentai.RawDocument(content=image_content, mime_type=mime_type)
# Configure the process request
request = documentai.ProcessRequest(
name=name, raw_document=raw_document, field_mask=field_mask
)
result = client.process_document(request=request)
# For a full list of `Document` object attributes, reference this page:
# https://cloud.google.com/document-ai/docs/reference/rest/v1/Document
document = result.document
# Read the text recognition output from the processor
with open("out.json", "w") as f:
print("The document contains the following text:")
print(document.entities, file=f)
if __name__ == "__main__":
process_document_sample(
"<project_id>",
"us",
"<processor_id>",
"<file_path>",
"image/jpeg",
None,
"<processor_version_id>"
)
コメントを残す