[A-00192] Rust入門
Rustプログラミングの入門ネタをまとめました。
Rustup,cargoのインストールは下記の記事を参考にしてください。
https://www.rust-lang.org/tools/install
・Hello,worldしてみる。
適当な場所でcargo(パッケージマネージャ)を使ってhelloworldプロジェクトを作成します。
cargo new helloworld
作成した環境は下記の通りです。
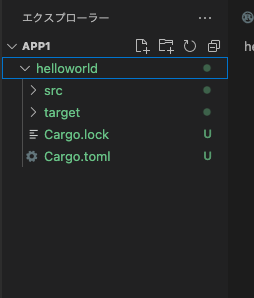
helloworldフォルダ直下に移動してbuildします。
cd helloworld
cargo build
$ cargo build
Finished dev [unoptimized + debuginfo] target(s) in 0.10s
下記のコマンドでプログラムを実行します。
./target/debug/helloworld
$ ./target/debug/helloworld
Hello, world!
rustプログラミングに慣れるため、main.rsを編集してみます。
fn main() {
println!("Hello, world!");
println!("Hello, Rust!");
}
再度cargoでbuildしてプログラムを実行すると下記の通りになります。
$ ./target/debug/helloworld
Hello, world!
Hello, Rust!
・構造体を作る
RustにはclassがありませんがC言語のようにstructという構造体宣言のようなものがあります。特徴としてプロパティと型の宣言しかできず、メソッドなどを持たせるということはできません。とりあえず作ってみます。
まずディレクトリ構成は下記となります。
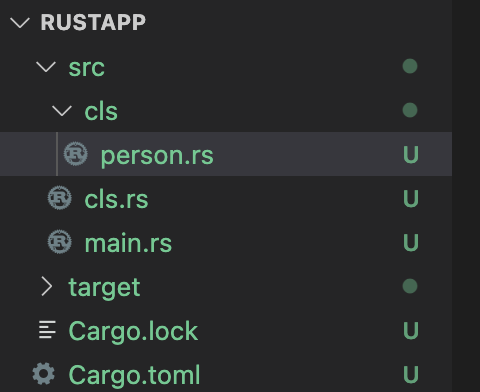
#[derive(Debug)]
pub struct Person {
pub name: String,
pub age: i8,
pub country: String
}
impl Person {
pub fn new(name:String, age: i8, country: String) -> Person {
Person { name: name
, age: age
, country: country}
}
}
pub use self::person::Person;
pub mod person;
mod cls;
fn main() {
println!("Hello, world!");
let p1 = cls::Person::new("satoshi".to_string(), 14, "Japan".to_string());
println!("{}", p1.name)
}
ビルドして実行します。
$ ./target/debug/rustapp
Hello, world!
satoshi
・Appendix
公式ドキュメントはこちら
コメントを残す