[A-00193] C++ 入門
c++の初心者向けの記事となります。
・とりあえずhelloworldしてみる
使用している端末はmacなのでclangのversionを確認します。
$ clang --version
Apple clang version 13.0.0 (clang-1300.0.29.30)
Target: x86_64-apple-darwin20.6.0
Thread model: posix
InstalledDir: /Library/Developer/CommandLineTools/usr/bin
ちょっとバージョン古い気がしますがとりあえず使えるのでhelloworldしてみます。
適当な場所でmain.cppファイルを作成します。
#include <iostream>
using namespace std;
int main() {
std::cout << "hello,world." << std::endl;
return 0;
}
上記を作成したら下記のコマンドを実行します。
clang++ main.cpp
$ clang++ main.cpp
$ ls
a.out main.cpp output
$ ./a.out
hello,world.
ちなみにoutputというフォルダがありますが、vscodeでrunした時にはoutputフォルダに実行ファイルを作成してくれます。
・クラスを作成してみる
C++にはクラスという概念がありますのでクラスを作ってみます。
ディレクトリ構造は下記の通りです。
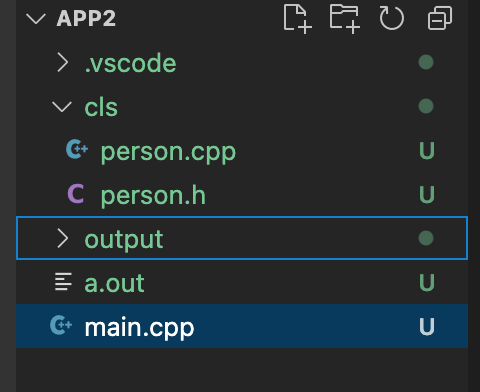
#ifndef _PERSON_H_
#define _PERSON_H_
#include <string>
using namespace std;
class Person
{
private:
std::string name;
int age;
std::string country;
public:
Person(std::string name, int age, std::string country);
~Person();
void sayHello();
};
Person::Person(std::string name, int age, std::string country)
{
Person::name = name;
Person::age = age;
Person::country = country;
}
Person::~Person()
{
}
#endif // !_PERSON_H_
#include "person.h"
#include <iostream>
using namespace std;
void Person::sayHello()
{
std::cout << "Hello! My name is " + Person::name + "!\n";
std::cout << "I'm " + std::to_string(Person::age) + " years old now.\n";
std::cout << "I'm live in " + Person::country + ".\n";
}
#include <iostream>
#include "cls/person.cpp"
using namespace std;
int main() {
Person p1("satoshi", 14, "Japan");
p1.sayHello();
return 0;
}
上記を実行すると下記のように動きます。
$ clang++ main.cpp
$ ls
a.out cls main.cpp output
$ ./a.out
Hello! My name is satoshi!
I'm 14 years old now.
I'm live in Japan.
・Appendix
参考文献はこちら
https://qiita.com/monhan/items/ea7e642b91392bfce8a6
https://qiita.com/niwasawa/items/d878eb7175ee9c3002be
https://cad.lolipop.jp/work/class/CaseInCPP11/CPPBasic/class_definition.htm
https://qiita.com/Yuya-Shimizu/items/45d42fe2942a684fa96a
コメントを残す