[A-00198] C#入門
C#の入門用記事です。
プロジェクトの作成などは全てdotnetを使用しますので.NETをインストールしておいてください。
・helloworldしてみる
コンソールアプリケーションを作成するので下記のコマンドでプロジェクトを作成します。
dotnet new console
実行完了すると下記のような構造ができます。
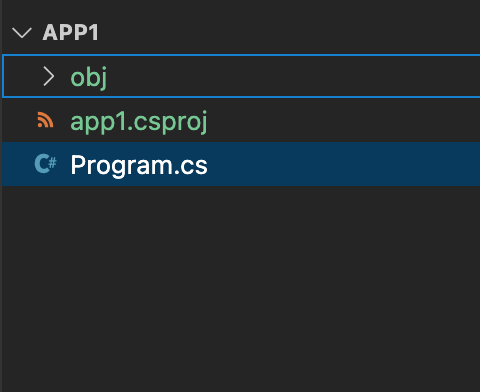
自動で作成されたProgram.csをビルドしてみます。
dotnet build
次に実行します。
$ dotnet run
Hello, World!
・クラスを作成してみる
次にクラスを作成して実行してみます。
ディレクトリ構造は下記の通り
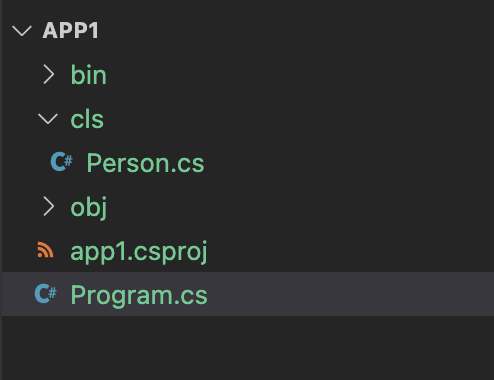
public class Person {
private string name;
private int age;
private string country;
public Person(string name, int age, string country) {
this.name = name;
this.age = age;
this.country = country;
}
public void sayHello() {
Console.WriteLine("Hello, my name is " + this.name + ".");
Console.WriteLine("I'm " + this.age + " years old now.");
Console.WriteLine("I'm live in " + this.country + ".");
}
}
using System;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
Person p = new Person("satoshi", 14, "Japan");
p.sayHello();
}
}
}
上記を作成したら下記のビルドして実行します。
$ dotnet build
MSBuild のバージョン 17.9.6+a4ecab324 (.NET)
復元対象のプロジェクトを決定しています...
復元対象のすべてのプロジェクトは最新です。
app1 -> ~/app1/bin/Debug/net8.0/app1.dll
ビルドに成功しました。
0 個の警告
0 エラー
経過時間 00:00:02.35
$ dotnet run
Hello, my name is satoshi.
I'm 14 years old now.
I'm live in Japan.
・インターフェースを作成してみる
次にインターフェースを作成してみます。
ディレクトリ構造は下記の通り
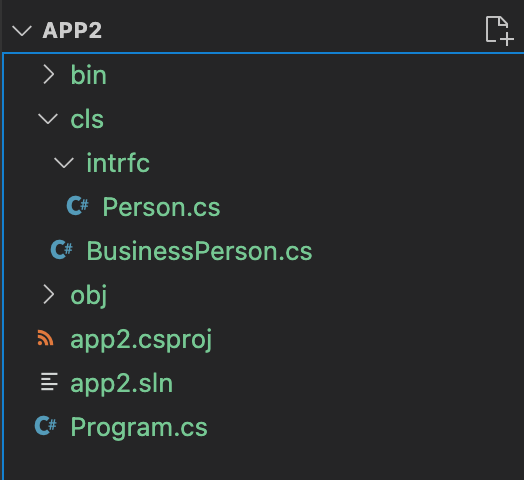
public interface Person {
void sayHello();
}
public class BusinessPerson : Person {
private string job {set; get;}
private string name { get; set; }
private int age { get; set; }
private string country { get; set; }
public BusinessPerson(string name, int age, string country
, string job) {
this.name = name;
this.age = age;
this.country = country;
this.job = job;
}
public void sayHello() {
Console.WriteLine("Hello, my name is " + this.name + "." );
Console.WriteLine("I'm " + this.age + " years old.");
Console.WriteLine("I'm work as " + this.job + " in " + this.country + " now.");
}
}
using System;
namespace ConsoleApp {
internal class Program {
public static void Main(string[] args) {
BusinessPerson bp = new BusinessPerson("satoshi", 14, "Japan", "Student");
bp.sayHello();
}
}
}
下記が実行結果です。
$ dotnet build
MSBuild のバージョン 17.9.6+a4ecab324 (.NET)
復元対象のプロジェクトを決定しています...
復元対象のすべてのプロジェクトは最新です。
app2 -> ~app2/bin/Debug/net8.0/app2.dll
ビルドに成功しました。
0 個の警告
0 エラー
経過時間 00:00:06.21
$ dotnet run
Hello, my name is satoshi.
I'm 14 years old.
I'm work as Student in Japan now.
・Appendix
参考文献はこちら
https://ufcpp.net/study/csharp/misc_autoimpl.html
https://qiita.com/yutorisan/items/d28386f168f2f3ab166d
https://qiita.com/haruka_kotani/items/c10227dc442e8396c3bb
https://atmarkit.itmedia.co.jp/fdotnet/csharp_abc/csharp_abc_003/csharp_abc02.html
コメントを残す