[A-00201] Java入門
javaの入門用記事です。基本的な動かし方を記載していきます。基本的にmavenを使用してプロジェクト形式でプログラムを作成します。
・helloworldしてみる
とりあえずhelloworldしてみます。
mvn archetype:generate \
-DarchetypeArtifactId=maven-archetype-quickstart \
-DinteractiveMode=false \
-DgroupId=example \
-DartifactId=helloworldapps
package example;
/**
* Hello world!
*
*/
public class App
{
public static void main( String[] args )
{
System.out.println( "Hello World!" );
}
}
jarファイルから実行したいのでMANIFEST.MFを作成したいと思います。
下記のようなディレクトリ構造にします。
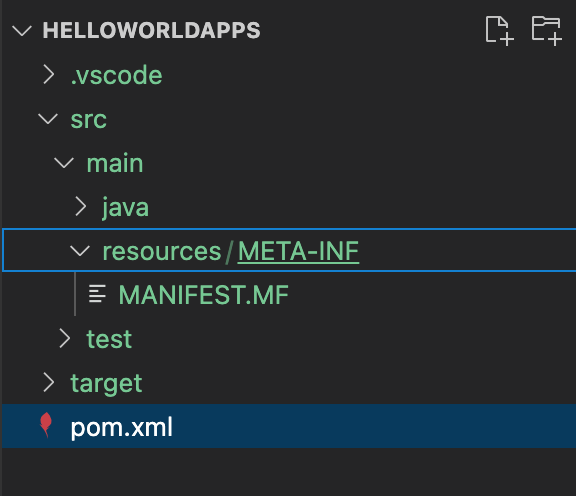
MANIFEST.MFの内容は下記の通りです。
Manifest-Version: 1.0
Main-Class: example.App
pom.xmlにpluginを定義します。
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>example</groupId>
<artifactId>helloworldapps</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<name>helloworldapps</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jar-plugin</artifactId>
<version>3.1.0</version>
<configuration>
<archive>
<manifestFile>${basedir}/src/main/resources/META-INF/MANIFEST.MF</manifestFile>
<manifestEntries>
<addDefaultSpecificationEntries>false</addDefaultSpecificationEntries>
<addDefaultImplementationEntries>false</addDefaultImplementationEntries>
</manifestEntries>
</archive>
</configuration>
</plugin>
</plugins>
</build>
</project>
上記を設定したのち、プロジェクト直下でmvnコマンドを実行します。
mvn clean install
サクセスで完了したら下記のコマンドを実行します。
java -jar target/helloworldapps-1.0-SNAPSHOT.jar
実行結果は下記の通り
$ java -jar target/helloworldapps-1.0-SNAPSHOT.jar
Hello World!
・クラスを作成してみる
次にクラスを作成してみます。 ディレクトリ構造は下記の通り
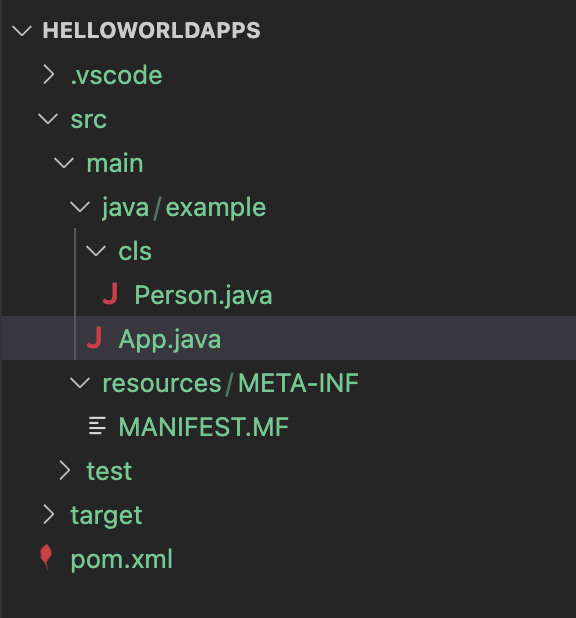
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>example</groupId>
<artifactId>helloworldapps</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<name>helloworldapps</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.32</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jar-plugin</artifactId>
<version>3.1.0</version>
<configuration>
<archive>
<manifestFile>${basedir}/src/main/resources/META-INF/MANIFEST.MF</manifestFile>
<manifestEntries>
<addDefaultSpecificationEntries>false</addDefaultSpecificationEntries>
<addDefaultImplementationEntries>false</addDefaultImplementationEntries>
</manifestEntries>
</archive>
</configuration>
</plugin>
</plugins>
</build>
</project>
package example.cls;
import lombok.Getter;
import lombok.Setter;
@Setter
@Getter
public class Person {
private String name;
private int age;
private String country;
public Person(String name, int age, String country) {
this.name = name;
this.age = age;
this.country = country;
}
public void sayHello() {
System.out.println("Hello, my name is " + this.name + ".");
System.out.println("I'm " + this.age + " years old.");
System.out.println("I'm live in " + this.country + ".");
}
}
package example;
import example.cls.Person;
/**
* Hello world!
*
*/
public class App
{
public static void main( String[] args )
{
System.out.println( "Hello World!" );
Person p = new Person("satoshi", 14, "Japan");
p.sayHello();
}
}
こちらが実行結果です。
$ java -jar target/helloworldapps-1.0-SNAPSHOT.jar
Hello World!
Hello, my name is satoshi.
I'm 14 years old.
I'm live in Japan.
・インターフェースを使ってみる
次にインターフェースを作成してみます。
package example.cls;
public interface Person {
void sayHello();
}
package example.cls;
import lombok.Getter;
import lombok.Setter;
@Setter
@Getter
public class BusinessPerson implements Person {
private String name;
private int age;
private String country;
public BusinessPerson(String name, int age, String country) {
this.name = name;
this.age = age;
this.country = country;
}
@Override
public void sayHello() {
System.out.println("My name is " + this.name + ".");
System.out.println("I'm " + this.age + " years old.");
System.out.println("I'm live in " + this.country + ".");
}
}
package example;
import example.cls.BusinessPerson;
/**
* Hello world!
*
*/
public class App
{
public static void main( String[] args )
{
System.out.println( "Hello World!" );
BusinessPerson p = new BusinessPerson("satoshi", 14, "Japan");
p.sayHello();
}
}
実行結果はこちら
$ java -jar target/helloworldapps-1.0-SNAPSHOT.jar
Hello World!
My name is satoshi.
I'm 14 years old.
I'm live in Japan.
コメントを残す